Python File in Read Mode Is Getting Changed
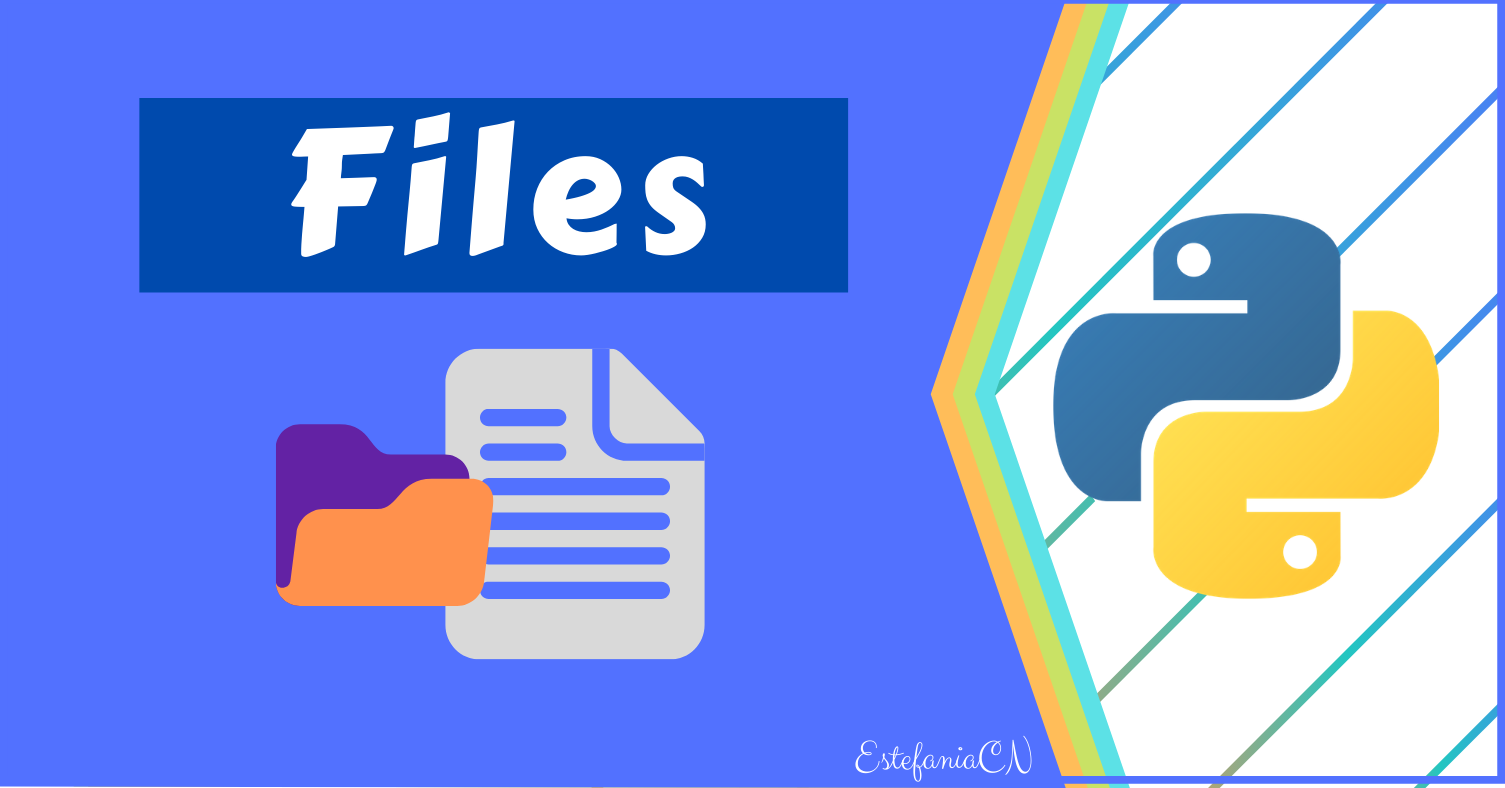
Welcome
Howdy! If you desire to learn how to work with files in Python, and so this article is for you lot. Working with files is an of import skill that every Python developer should acquire, so allow's get started.
In this commodity, you will learn:
- How to open a file.
- How to read a file.
- How to create a file.
- How to modify a file.
- How to close a file.
- How to open up files for multiple operations.
- How to piece of work with file object methods.
- How to delete files.
- How to piece of work with context managers and why they are useful.
- How to handle exceptions that could exist raised when you lot work with files.
- and more!
Let'southward begin! ✨
🔹 Working with Files: Basic Syntax
1 of the well-nigh important functions that you volition need to use as you piece of work with files in Python is open()
, a born role that opens a file and allows your program to utilize it and piece of work with it.
This is the bones syntax:
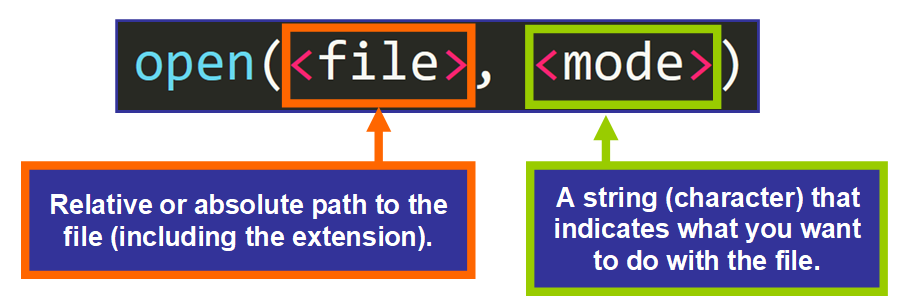
💡 Tip: These are the ii most commonly used arguments to phone call this function. There are six additional optional arguments. To acquire more virtually them, please read this article in the documentation.
Beginning Parameter: File
The starting time parameter of the open up()
part is file
, the absolute or relative path to the file that yous are trying to piece of work with.
We unremarkably utilize a relative path, which indicates where the file is located relative to the location of the script (Python file) that is calling the open up()
office.
For example, the path in this function call:
open("names.txt") # The relative path is "names.txt"
Only contains the name of the file. This can be used when the file that you are trying to open is in the aforementioned directory or binder as the Python script, similar this:

But if the file is within a nested binder, like this:

Then nosotros demand to use a specific path to tell the function that the file is within another folder.
In this example, this would be the path:
open("information/names.txt")
Find that we are writing data/
beginning (the proper name of the folder followed by a /
) and then names.txt
(the name of the file with the extension).
💡 Tip: The three letters .txt
that follow the dot in names.txt
is the "extension" of the file, or its blazon. In this example, .txt
indicates that information technology's a text file.
Second Parameter: Mode
The second parameter of the open up()
function is the mode
, a string with one character. That single character basically tells Python what you are planning to do with the file in your program.
Modes available are:
- Read (
"r"
). - Suspend (
"a"
) - Write (
"w"
) - Create (
"10"
)
You can also choose to open the file in:
- Text style (
"t"
) - Binary mode (
"b"
)
To use text or binary mode, you lot would need to add these characters to the primary mode. For example: "wb"
means writing in binary mode.
💡 Tip: The default modes are read ("r"
) and text ("t"
), which means "open for reading text" ("rt"
), so you don't need to specify them in open()
if you want to use them considering they are assigned by default. You tin can only write open(<file>)
.
Why Modes?
It really makes sense for Python to grant only sure permissions based what you are planning to do with the file, right? Why should Python permit your program to practice more than necessary? This is basically why modes exist.
Think about it — allowing a program to do more than necessary can problematic. For example, if yous but need to read the content of a file, it can be dangerous to allow your plan to modify information technology unexpectedly, which could potentially introduce bugs.
🔸 How to Read a File
Now that you know more about the arguments that the open()
office takes, let'due south see how y'all can open a file and store it in a variable to use it in your program.
This is the basic syntax:

We are simply assigning the value returned to a variable. For example:
names_file = open("data/names.txt", "r")
I know y'all might be asking: what type of value is returned past open()
?
Well, a file object.
Permit's talk a niggling bit about them.
File Objects
According to the Python Documentation, a file object is:
An object exposing a file-oriented API (with methods such as read() or write()) to an underlying resource.
This is basically telling us that a file object is an object that lets us work and interact with existing files in our Python program.
File objects have attributes, such equally:
- proper name: the name of the file.
- closed:
True
if the file is closed.False
otherwise. - fashion: the style used to open the file.
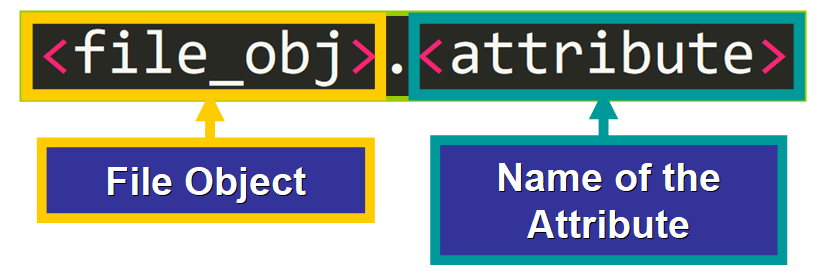
For example:
f = open up("data/names.txt", "a") print(f.mode) # Output: "a"
Now let'south see how you tin can admission the content of a file through a file object.
Methods to Read a File
For us to exist able to work file objects, nosotros need to take a way to "interact" with them in our program and that is exactly what methods exercise. Let's see some of them.
Read()
The first method that yous need to learn nearly is read()
, which returns the entire content of the file equally a string.

Here nosotros take an case:
f = open up("data/names.txt") print(f.read())
The output is:
Nora Gino Timmy William
Y'all can use the type()
office to ostend that the value returned past f.read()
is a string:
print(type(f.read())) # Output <class 'str'>
Yeah, it's a string!
In this case, the entire file was printed because we did non specify a maximum number of bytes, but we can do this also.
Hither we have an case:
f = open("information/names.txt") print(f.read(3))
The value returned is limited to this number of bytes:
Nor
❗️Of import: You need to close a file after the chore has been completed to free the resources associated to the file. To do this, you need to telephone call the close()
method, similar this:

Readline() vs. Readlines()
You tin read a file line by line with these two methods. They are slightly different, so allow's see them in detail.
readline()
reads 1 line of the file until information technology reaches the end of that line. A trailing newline grapheme (\n
) is kept in the string.
💡 Tip: Optionally, you can pass the size, the maximum number of characters that y'all want to include in the resulting cord.
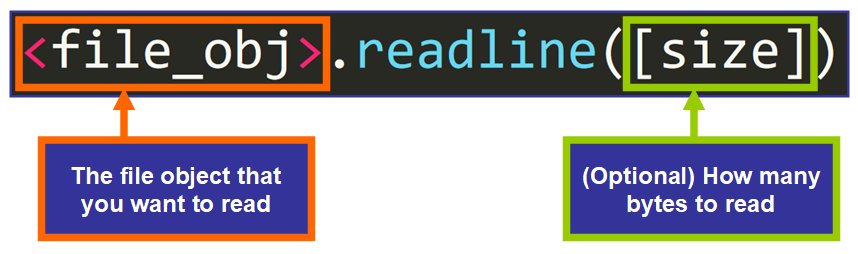
For instance:
f = open("data/names.txt") impress(f.readline()) f.shut()
The output is:
Nora
This is the first line of the file.
In contrast, readlines()
returns a list with all the lines of the file every bit private elements (strings). This is the syntax:

For example:
f = open("data/names.txt") print(f.readlines()) f.shut()
The output is:
['Nora\n', 'Gino\north', 'Timmy\due north', 'William']
Discover that in that location is a \n
(newline character) at the end of each cord, except the final one.
💡 Tip: Y'all can get the same list with listing(f)
.
Yous can piece of work with this list in your program by assigning it to a variable or using it in a loop:
f = open("data/names.txt") for line in f.readlines(): # Exercise something with each line f.close()
We tin besides iterate over f
directly (the file object) in a loop:
f = open("data/names.txt", "r") for line in f: # Do something with each line f.close()
Those are the master methods used to read file objects. Now permit's run across how you tin create files.
🔹 How to Create a File
If you need to create a file "dynamically" using Python, you can do it with the "x"
mode.
Let'southward see how. This is the basic syntax:

Here's an case. This is my current working directory:

If I run this line of code:
f = open("new_file.txt", "x")
A new file with that name is created:

With this mode, you can create a file and then write to it dynamically using methods that y'all will learn in just a few moments.
💡 Tip: The file will exist initially empty until y'all modify information technology.
A curious matter is that if you effort to run this line again and a file with that proper noun already exists, you volition see this error:
Traceback (most recent call last): File "<path>", line 8, in <module> f = open up("new_file.txt", "10") FileExistsError: [Errno 17] File exists: 'new_file.txt'
According to the Python Documentation, this exception (runtime error) is:
Raised when trying to create a file or directory which already exists.
Now that you know how to create a file, let's see how you tin modify it.
🔸 How to Modify a File
To modify (write to) a file, y'all demand to employ the write()
method. You have two ways to exercise it (suspend or write) based on the fashion that you choose to open it with. Let'due south see them in detail.
Append
"Appending" means calculation something to the finish of another affair. The "a"
way allows you to open up a file to append some content to it.
For case, if nosotros accept this file:
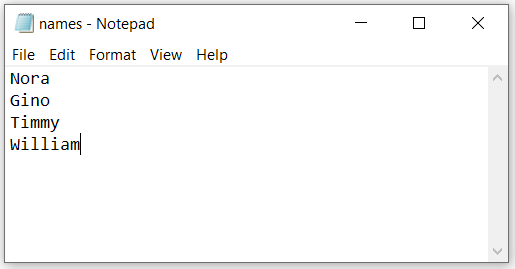
And nosotros want to add a new line to it, we tin open it using the "a"
way (append) and then, call the write()
method, passing the content that we want to suspend as statement.
This is the basic syntax to call the write()
method:

Hither's an example:
f = open("data/names.txt", "a") f.write("\nNew Line") f.close()
💡 Tip: Notice that I'm calculation \n
before the line to indicate that I want the new line to appear as a carve up line, not every bit a continuation of the existing line.
This is the file at present, later running the script:

💡 Tip: The new line might not be displayed in the file until f.close()
runs.
Write
Sometimes, you may want to delete the content of a file and replace it entirely with new content. You can do this with the write()
method if you open the file with the "w"
manner.
Here we take this text file:
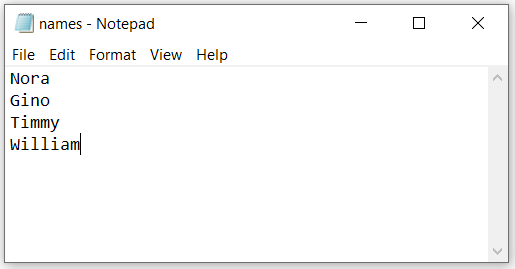
If I run this script:
f = open up("data/names.txt", "west") f.write("New Content") f.close()
This is the result:
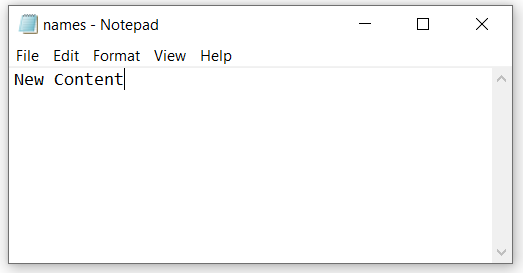
Every bit yous can see, opening a file with the "due west"
mode and then writing to it replaces the existing content.
💡 Tip: The write()
method returns the number of characters written.
If you want to write several lines at once, you tin use the writelines()
method, which takes a list of strings. Each string represents a line to exist added to the file.
Here's an case. This is the initial file:
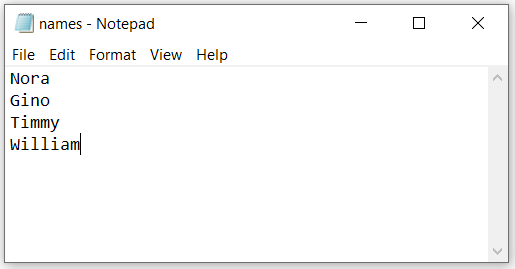
If nosotros run this script:
f = open up("data/names.txt", "a") f.writelines(["\nline1", "\nline2", "\nline3"]) f.shut()
The lines are added to the finish of the file:
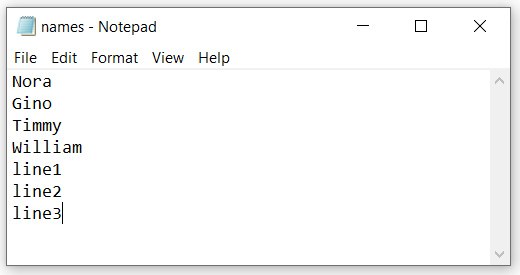
Open File For Multiple Operations
Now you know how to create, read, and write to a file, but what if you want to do more than than i affair in the same program? Let's see what happens if nosotros try to do this with the modes that yous have learned and then far:
If you open a file in "r"
fashion (read), and then try to write to it:
f = open("information/names.txt") f.write("New Content") # Trying to write f.shut()
Yous will get this mistake:
Traceback (most recent call last): File "<path>", line 9, in <module> f.write("New Content") io.UnsupportedOperation: non writable
Similarly, if y'all open a file in "westward"
manner (write), and so endeavor to read it:
f = open("data/names.txt", "w") print(f.readlines()) # Trying to read f.write("New Content") f.close()
Yous will encounter this fault:
Traceback (most recent call last): File "<path>", line 14, in <module> print(f.readlines()) io.UnsupportedOperation: not readable
The same will occur with the "a"
(suspend) style.
How tin we solve this? To be able to read a file and perform another operation in the same program, y'all need to add the "+"
symbol to the mode, similar this:
f = open("data/names.txt", "west+") # Read + Write
f = open up("data/names.txt", "a+") # Read + Append
f = open("information/names.txt", "r+") # Read + Write
Very useful, correct? This is probably what yous will employ in your programs, but exist certain to include merely the modes that you need to avoid potential bugs.
Sometimes files are no longer needed. Let's see how you can delete files using Python.
🔹 How to Delete Files
To remove a file using Python, you need to import a module called bone
which contains functions that collaborate with your operating system.
💡 Tip: A module is a Python file with related variables, functions, and classes.
Particularly, y'all need the remove()
function. This function takes the path to the file as argument and deletes the file automatically.

Let's encounter an example. We desire to remove the file called sample_file.txt
.

To exercise it, nosotros write this code:
import os os.remove("sample_file.txt")
- The beginning line:
import os
is called an "import statement". This statement is written at the top of your file and it gives you access to the functions divers in theos
module. - The second line:
os.remove("sample_file.txt")
removes the file specified.
💡 Tip: you tin can use an absolute or a relative path.
Now that you know how to delete files, permit'southward see an interesting tool... Context Managers!
🔸 Meet Context Managers
Context Managers are Python constructs that will make your life much easier. Past using them, y'all don't need to recall to close a file at the end of your plan and you take access to the file in the item office of the programme that you choose.
Syntax
This is an instance of a context managing director used to work with files:

💡 Tip: The body of the context manager has to be indented, only like nosotros indent loops, functions, and classes. If the code is not indented, it will not exist considered part of the context managing director.
When the body of the context manager has been completed, the file closes automatically.
with open("<path>", "<mode>") every bit <var>: # Working with the file... # The file is closed here!
Example
Here's an example:
with open("data/names.txt", "r+") equally f: print(f.readlines())
This context director opens the names.txt
file for read/write operations and assigns that file object to the variable f
. This variable is used in the torso of the context manager to refer to the file object.
Trying to Read it Once more
Later on the body has been completed, the file is automatically closed, and so information technology tin't be read without opening it again. But wait! We have a line that tries to read it again, right hither below:
with open("data/names.txt", "r+") as f: print(f.readlines()) print(f.readlines()) # Trying to read the file again, outside of the context manager
Permit's see what happens:
Traceback (most recent call final): File "<path>", line 21, in <module> impress(f.readlines()) ValueError: I/O operation on closed file.
This error is thrown because nosotros are trying to read a closed file. Awesome, right? The context manager does all the heavy work for us, it is readable, and concise.
🔹 How to Handle Exceptions When Working With Files
When y'all're working with files, errors can occur. Sometimes you may non have the necessary permissions to modify or access a file, or a file might not even exist.
As a developer, you need to foresee these circumstances and handle them in your programme to avoid sudden crashes that could definitely affect the user experience.
Let'southward see some of the most mutual exceptions (runtime errors) that y'all might find when y'all work with files:
FileNotFoundError
According to the Python Documentation, this exception is:
Raised when a file or directory is requested but doesn't exist.
For example, if the file that y'all're trying to open doesn't exist in your current working directory:
f = open up("names.txt")
Y'all will see this error:
Traceback (most recent phone call last): File "<path>", line eight, in <module> f = open("names.txt") FileNotFoundError: [Errno two] No such file or directory: 'names.txt'
Allow's interruption this error down this line past line:
-
File "<path>", line 8, in <module>
. This line tells you that the error was raised when the code on the file located in<path>
was running. Specifically, whenline eight
was executed in<module>
. -
f = open up("names.txt")
. This is the line that caused the mistake. -
FileNotFoundError: [Errno 2] No such file or directory: 'names.txt'
. This line says that aFileNotFoundError
exception was raised because the file or directorynames.txt
doesn't exist.
💡 Tip: Python is very descriptive with the fault messages, right? This is a huge advantage during the procedure of debugging.
PermissionError
This is another mutual exception when working with files. According to the Python Documentation, this exception is:
Raised when trying to run an operation without the adequate access rights - for instance filesystem permissions.
This exception is raised when yous are trying to read or modify a file that don't have permission to admission. If yous effort to practice and so, you lot will see this error:
Traceback (near recent call final): File "<path>", line 8, in <module> f = open up("<file_path>") PermissionError: [Errno 13] Permission denied: 'data'
IsADirectoryError
Co-ordinate to the Python Documentation, this exception is:
Raised when a file performance is requested on a directory.
This particular exception is raised when you effort to open or piece of work on a directory instead of a file, so be really careful with the path that you pass as statement.
How to Handle Exceptions
To handle these exceptions, you tin can employ a endeavour/except statement. With this statement, you can "tell" your programme what to do in case something unexpected happens.
This is the bones syntax:
effort: # Try to run this code except <type_of_exception>: # If an exception of this type is raised, end the procedure and jump to this cake
Hither you tin see an case with FileNotFoundError
:
endeavor: f = open("names.txt") except FileNotFoundError: print("The file doesn't exist")
This basically says:
- Try to open up the file
names.txt
. - If a
FileNotFoundError
is thrown, don't crash! Merely impress a descriptive statement for the user.
💡 Tip: Yous can choose how to handle the situation by writing the appropriate code in the except
block. Perhaps y'all could create a new file if it doesn't exist already.
To close the file automatically afterwards the task (regardless of whether an exception was raised or not in the try
cake) you tin can add the finally
block.
try: # Try to run this lawmaking except <exception>: # If this exception is raised, terminate the process immediately and leap to this cake finally: # Practise this after running the code, even if an exception was raised
This is an example:
try: f = open("names.txt") except FileNotFoundError: print("The file doesn't be") finally: f.shut()
There are many ways to customize the try/except/finally statement and you tin even add together an else
cake to run a block of lawmaking only if no exceptions were raised in the try
cake.
💡 Tip: To acquire more nearly exception treatment in Python, you may like to read my article: "How to Handle Exceptions in Python: A Detailed Visual Introduction".
🔸 In Summary
- Y'all can create, read, write, and delete files using Python.
- File objects have their own set of methods that yous can utilize to work with them in your program.
- Context Managers assist y'all piece of work with files and manage them past closing them automatically when a task has been completed.
- Exception treatment is key in Python. Mutual exceptions when yous are working with files include
FileNotFoundError
,PermissionError
andIsADirectoryError
. They tin exist handled using endeavour/except/else/finally.
I really hope you liked my article and found it helpful. Now you can work with files in your Python projects. Bank check out my online courses. Follow me on Twitter. ⭐️
Learn to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-write-to-file-open-read-append-and-other-file-handling-functions-explained/
0 Response to "Python File in Read Mode Is Getting Changed"
Post a Comment